Web of Things Arduino: Matrix display
A Matrix display connected to an ESP32. It exposes a HTTP REST API in order to accept text to display.
Prerequisites #
- Windows, MacOS, Linux
- ESP32
- Installed Arduino IDE with Web of Things library
- WiFi Router
- MAX7219 Dot Matrix display module 4x8x8
- Female to female DuPont jumper cable
- Installed Insomnia or another REST Client
Before you dive into the tutorial, you should read more about Web of Things in order to understand what some terms mean
Connect your ESP32 to the Matrix display #
ESP32 Pin | MAX7219 Matrix | |
---|---|---|
VCC (3.3V) | → | VCC (VDD) |
GND | → | GND |
D23 (IO23) | → | DIN (MOSI) |
D5 (IO5) | → | CS (SS) |
D18 (IO18) | → | CLK |
Open a new project in the Arduino IDE #
Create a new project under the menu point File → New
in the Arduino IDE.
Install dependencies #
In order to drive the Matrix display we need two libraries.
The first one is MD_MAX72XX
, and the second one is MD_Parola
.
You can install those libraries over the Library Manager in the Arduino IDE.
Just open it over the menu Tools → Manage Libraries...
.
Copy the app code #
Copy the Matrix display code into your open main file in the Arduino IDE.
Change the configuration #
In order to be able to connect to your router, you need to change the values of the variables ssid
and password
in the copied app code.
Flash the code #
Before you flash the code, you should open the serial monitor under the menu point Tools → Serial Monitor
.
You should now flash the code under Sketch → Upload
.
You can also use the Upload
button in the Arduino IDE.
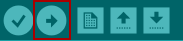
When the flashing is done, the ESP32 should restart.
If this doesn’t happen, push the EN
button yourself.
The ESP32 should blink while trying to connect to your WiFi router.
When the connection is successful the light should be constant instead of blinking.
You should be able to see the IP address of the ESP32 in the Serial Monitor.
Copy this IP address, we need it to access the REST API.
Test the display #
Get WoT TD
Create a new collection in your REST Client, if possible.
Add a new HTTP GET Request to it. Give it some meaningful name, such as Get TD
.
Use http://[YOUR_IP]/.well-known/wot-thing-description
as URL.
You have to replace [YOUR_IP]
with the IP address of your ESP32.
You previously opened the serial monitor in the Arduino IDE.
The ESP32 should have logged his own IP address in there.
Once you send the request, the ESP32 should respond with a valid Web of Things Thing Description.
The WoT TD should look similar to this one
{
"id": "matrixDisplay",
"title": "Matrix Display",
"@context": [
"https://www.w3.org/2019/wot/td/v1"
],
"description": "A web connected matrix display",
"base": "http://192.168.178.28/",
"securityDefinitions": {
"nosec_sc": {
"scheme": "nosec"
}
},
"security": "nosec_sc",
"@type": [
"Matrix Display"
],
"actions": {
"display": {
"title": "Display text",
"description": "display a text on a Matrix display",
"@type": "displayAction",
"input": {
"type": "object",
"properties": {
"body": {
"type": "string"
}
}
},
"forms": [
{
"href": "/things/matrixDisplay/actions/display"
}
]
}
},
"href": "/things/matrixDisplay"
}
The WoT TD describes the whole device and all available APIs.
In this case we only have one ActionAffordance
with the name display
available.
Test the ActionAffordance
The ActionAffordance
with the name display
is available under the endpoint /things/matrixDisplay/actions/display
.
You are going to send a test request to this endpoint.
Create another request in your REST Client. Change it to a POST
Request.
Give it a name such as display text
. You have to use http://[YOUR_IP]/things/matrixDisplay/actions/display
as URL.
As before, replace [YOUR_IP]
with the IP address of your ESP32.
The input of the ActionAffordance
is defined as the following
{
"input": {
"type": "object",
"properties": {
"body": {
"type": "string"
}
}
}
}
That means we have to use a JSON object for the body of the HTTP POST Request.
This JSON object has one property called body
which is of type string
.
Therefore the body of our test request is going to be the following:
{
"body": "99999"
}
When you send the request, there should be 99999 displayed on your matrix display. The ESP32 should also respond with a JSON similar to this one
{
"display": {
"input": {
"body": "99999"
},
"status": "created",
"timeRequested": "1970-01-01T00:00:00+00:00",
"href": "/things/matrixDisplay/actions/display/dcd5c1bcca5047c9"
}
}
Congratulations, your ESP32 with matrix works. In another tutorial we use the matrix LED display to show the number of stars and forks of our favourite Github repository. You may want to checkout this tutorial as well.